不辞鶗鴂妒年芳,但惜流尘暗烛房。
昨夜西池凉露满,桂花吹断月中香。
CABasicAnimation
- CABasicAnimation是核心动画类簇中的一个类,是
CAPropertyAnimation
的子类,它的祖父是CAAnimation
。它主要用于制作比较单一的动画,例如,平移、缩放、旋转、颜色渐变、边框的值的变化等,也就是将layer的某个属性值从一个值到另一个值的变化。 - 属性说明:
fromValue:
keyPath相应属性的初始值toValue:
keyPath相应属性的结束值
- 随着动画的进行,在长度为
duration
的持续时间内,keyPath相应属性的值从fromValue渐渐地变为toValue。 - 如果
fillMode=kCAFillModeForwards
和removedOnComletion=NO
,那么在动画执行完毕后,图层会保持显示动画执行后的状态。 - 但在实质上,图层的属性值还是动画执行前的初始值,并没有真正被改变。
- 比如,CALayer的
position
初始值为(0,0)
,CABasicAnimation的fromValue为(10,10)
,toValue为(100,100)
,虽然动画执行完毕后图层保持在(100,100)
这个位置,实质上图层的position还是为(0,0)。
keyPath(官方文档截图)
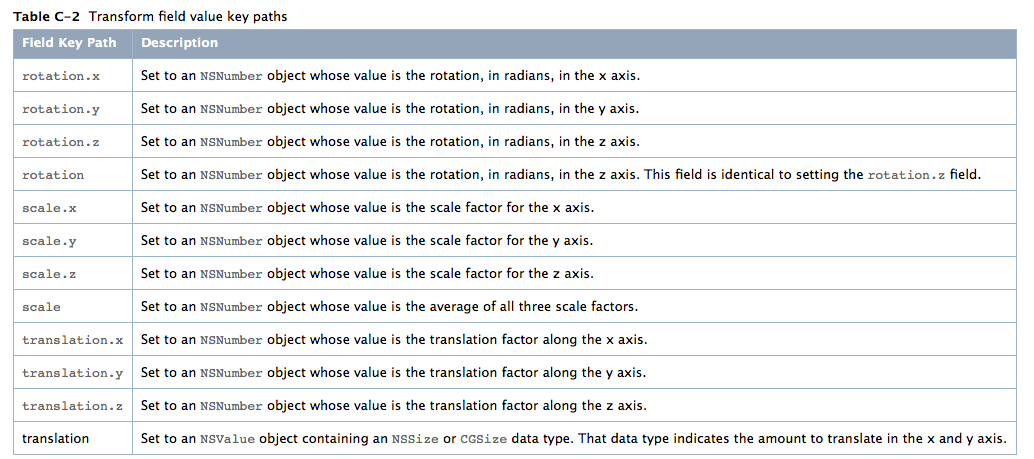
3D的形变属性
- 在文档的
CATransform3D key paths
这个位置查看keyPath的属性和包装的数值。
1 | /** X Y 轴的动画 |
位置属性的基本动画
1 | /** 位置属性的基本动画 |
旋转属性的基本动画
1 | /** 旋转属性的基本动画 |
缩放属性的基本动画
1 | /** 缩放属性的基本动画 |
keyFrameAnimation (关键帧动画)
- keyFrameAnimation是CApropertyAnimation的子类,跟CABasicAnimation的区别是:
CABasicAnimation
只能从一个数值(fromValue)变到另一个数值(toValue)- 而CAKeyframeAnimation会使用一个NSArray保存这些数值
- 属性说明:
values
:就是上述的NSArray对象。里面的元素称为”关键帧”(keyframe)。动画对象会在指定的时间(duration)内,依次显示values数组中的每一个关键帧path
:可以设置一个CGPathRef\CGMutablePathRef,让层跟着路径移动。path只对CALayer的anchorPoint和position起作用。如果你设置了path,那么values将被忽略。keyTimes
:可以为对应的关键帧指定对应的时间点,其取值范围为0到1.0。keyTimes中的每一个时间值都对应values中的每一帧.当keyTimes没有设置的时候,各个关键帧的时间是平分的。CABasicAnimation
可看做是最多只有2个关键帧的CAKeyframeAnimation。
帧动画的基本使用
1 | - (void)demo |
animation.path方法
1 | /** 让view沿着绘制的路径执行动画 |
图标抖动(模仿App删除)
1 | - (IBAction)star |
CATransition
- CAAnimation的子类,用于做转场动画,能够为层提供移出屏幕和移入屏幕的动画效果。iOS比Mac OS X的转场动画效果少一点。
- UINavigationController就是通过CATransition实现了将控制器的视图推入屏幕的动画效果。
- 属性解析:
type
:动画过渡类型subtype
:动画过渡方向startProgress
:动画起点(在整体动画的百分比)endProgress
:动画终点(在整体动画的百分比)
转场动画过渡效果
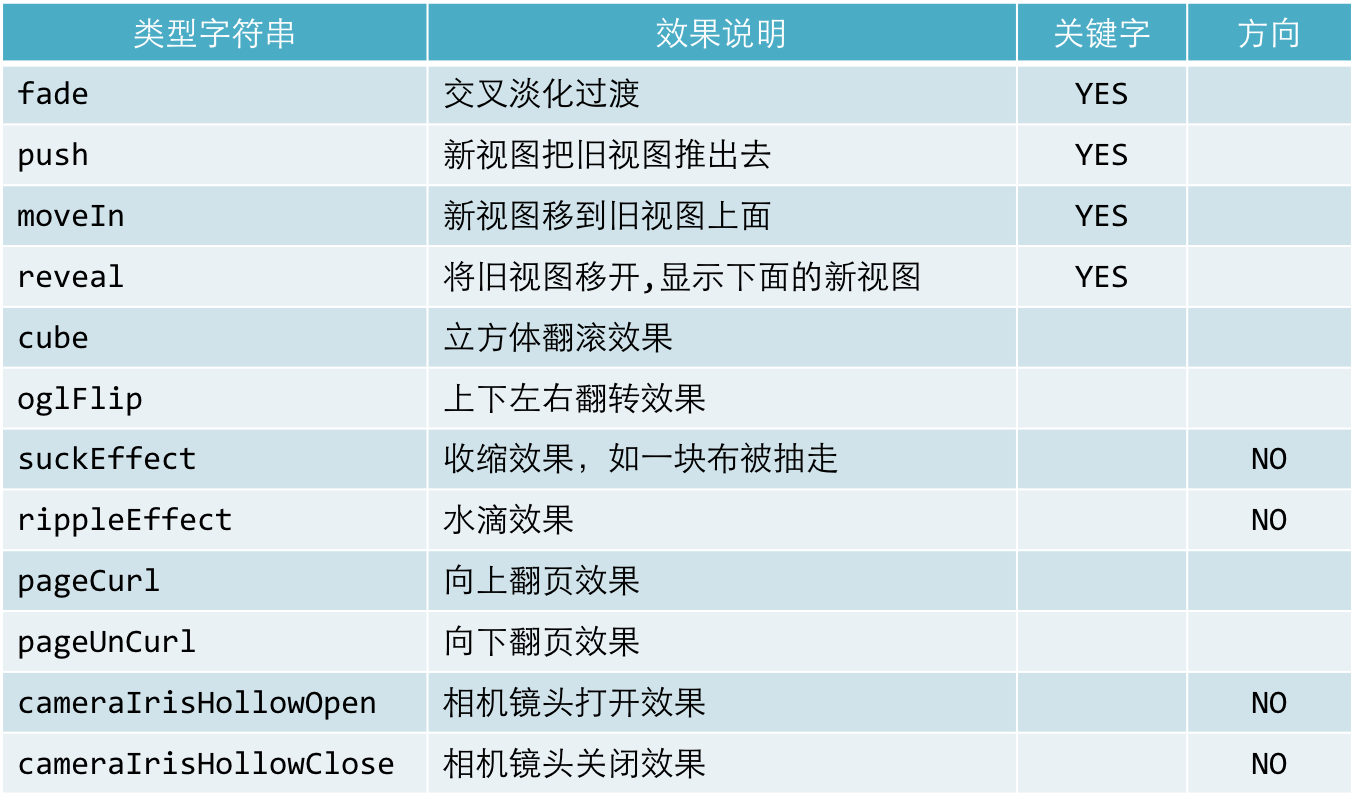
CAAnimationGroup
- CAAnimation的子类,可以保存一组动画对象,将CAAnimationGroup对象加入层后,组中所有动画对象可以同时并发运行。
- 属性解析:
animations
:用来保存一组动画对象的NSArray。- 默认情况下,一组动画对象是同时运行的,也可以通过设置动画对象的beginTime属性来更改动画的开始时间。
1 | // 将多个核心动画放在动画组里面一起执行 |
UIView动画封装
- UIKit直接将动画集成到UIView类中,当内部的一些属性发生改变时,UIView将为这些改变提供动画支持。
执行动画所需要的工作由UIView类自动完成,但仍要在希望执行动画时通知视图,为此需要将改变属性的代码放在
[UIView beginAnimations:nil context:nil]
和[UIView commitAnimations]
之间。常见方法解析:
1 | // 设置动画代理对象,当动画开始或者结束时会发消息给代理对象 |
Block动画
动画1
duration
:动画的持续时间delay
:动画延迟delay秒后开始options
:动画的节奏控制animations
:将改变视图属性的代码放在这个block中completion
:动画结束后,会自动调用这个block
1 | + (void)animateWithDuration:(NSTimeInterval)duration delay:(NSTimeInterval)delay options:(UIViewAnimationOptions)options animations:(void (^)(void))animations completion:(void (^)(BOOL finished))completion |
动画2
duration
:动画的持续时间view
:需要进行转场动画的视图options
:转场动画的类型animations
:将改变视图属性的代码放在这个block中completion
:动画结束后,会自动调用这个block
1 | + (void)transitionWithView:(UIView *)view duration:(NSTimeInterval)duration options:(UIViewAnimationOptions)options animations:(void (^)(void))animations completion:(void (^)(BOOL finished))completion |
动画3
duration
:动画的持续时间options
:转场动画的类型animations
:将改变视图属性的代码放在这个block中completion
:动画结束后,会自动调用这个block
1 | + (void)transitionFromView:(UIView *)fromView toView:(UIView *)toView duration:(NSTimeInterval)duration options:(UIViewAnimationOptions)options completion:(void (^)(BOOL finished))completion |
- 方法调用完毕后,
相当于执行了
下面两句代码:
1 | // 添加toView到父视图 |
UIImageView的帧动画
- UIImageView可以让一系列的图片在特定的时间内按顺序显示。
- 相关属性解析:
- animationImages:要显示的图片(一个装着UIImage的NSArray)。
- animationDuration:完整地显示一次animationImages中的所有图片所需的时间。
- animationRepeatCount:动画的执行次数(默认为0,代表无限循环)。
- 相关方法解析:
1 | - (void)startAnimating; 开始动画 |
UIActivityIndicatorView
- 是一个旋转进度轮,可以用来告知用户有一个操作正在进行中,一般用
initWithActivityIndicatorStyle
初始化。 UIActivityIndicatorViewStyle有3个值可供选择:
UIActivityIndicatorViewStyleWhiteLarge
:大型白色指示器UIActivityIndicatorViewStyleWhite
:标准尺寸白色指示器UIActivityIndicatorViewStyleGray
:灰色指示器,用于白色背景
方法解析:
1 | - (void)startAnimating; 开始动画 |